Public Member Functions |
Public Attributes |
Protected Member Functions |
Private Types |
Private Member Functions |
Private Attributes |
List of all members
Block::SpinBase Class Reference
Detailed Description
Base class for the implementation of a block of spin sites.
Definition at line 79 of file DMRGBlock.hpp.
#include <DMRGBlock.hpp>
Collaboration diagram for Block::SpinBase:
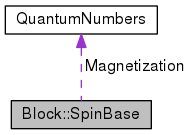
Public Member Functions | |
virtual PetscInt | loc_dim () const |
Local dimension of a single site. More... | |
virtual std::vector< PetscScalar > | loc_qn_list () const |
Sz sectors of a single site. More... | |
virtual std::vector< PetscInt > | loc_qn_size () const |
Number of states in each sector in a single site. More... | |
virtual PetscErrorCode | MatSpinSzCreate (Mat &Sz) |
Creates the single-site \( S^z \) operator. More... | |
virtual PetscErrorCode | MatSpinSpCreate (Mat &Sp) |
Creates the single-site raising operator \( S^+ \), from which we can define \( S^- = (S^+)^\dagger \). More... | |
PetscErrorCode | Initialize (const MPI_Comm &comm_in) |
Initializes block object's MPI attributes. More... | |
PetscErrorCode | Initialize (const MPI_Comm &comm_in, const PetscInt &num_sites_in, const PetscInt &num_states_in, const PetscBool &init_ops=PETSC_TRUE) |
Initializes block object with input attributes and array of matrix operators. More... | |
PetscErrorCode | Initialize (const MPI_Comm &comm_in, const PetscInt &num_sites_in, const std::vector< PetscReal > &qn_list_in, const std::vector< PetscInt > &qn_size_in, const PetscBool &init_ops=PETSC_TRUE) |
Initializes block object with input attributes and array of matrix operators. More... | |
PetscErrorCode | Initialize (const PetscInt &num_sites_in, const QuantumNumbers &qn_in) |
Initializes block object with an initialized quantum numbers object. More... | |
PetscErrorCode | InitializeFromDisk (const MPI_Comm &comm_in, const std::string &block_path) |
Initializes block object from data located in the directory block_path . More... | |
PetscErrorCode | InitializeSave (const std::string &save_dir_in) |
Initializes the writing of the block matrices to file. More... | |
PetscErrorCode | SetDiskStorage (const std::string &read_dir_in, const std::string &write_dir_in) |
Tells where to read from and save the operators and data about the block. More... | |
std::string | SaveDir () const |
Returns the value of save_dir where the block data will be read from/written. More... | |
PetscBool | SaveInitialized () const |
Tells whether InitializeSave() has been properly called. More... | |
PetscErrorCode | SaveAndDestroy () |
Save all the matrix operators to file and destroy the current storage. More... | |
PetscErrorCode | SaveBlockInfo () |
Save some information about the block that could be used to reconstruct it later. More... | |
PetscErrorCode | RetrieveOperator (const std::string &OpName, const size_t &isite, Mat &Op, const MPI_Comm &comm_in=MPI_COMM_NULL) |
Retrieves a single operator. More... | |
PetscErrorCode | Retrieve () |
Retrieve all the matrix operators that were written to file by SaveAndDestroy() More... | |
PetscErrorCode | EnsureSaved () |
Ensures that the block matrices have been saved if the block is initialized, otherwise does nothing. More... | |
PetscErrorCode | EnsureRetrieved () |
Ensures that the block matrices have been retrieved if the block is initialized, otherwise does nothing. More... | |
PetscErrorCode | Destroy () |
Destroys all operator matrices and frees memory. More... | |
PetscErrorCode | CheckOperatorArray (const Op_t &OpType) const |
Determines whether the operator arrays have been successfully filled with matrices. More... | |
PetscBool | Initialized () const |
Indicates whether block has been initialized before using it. More... | |
PetscBool | Saved () const |
Returns the saved state of all operators. More... | |
MPI_Comm | MPIComm () const |
Gets the communicator associated to the block. More... | |
PetscInt | NumSites () const |
Gets the number of sites that are currently initialized. More... | |
PetscInt | NumStates () const |
Gets the number of states that are currently used. More... | |
Mat | Sz (const PetscInt &Isite) const |
Returns the matrix pointer to the \( S^z \) operator at site Isite . More... | |
Mat | Sp (const PetscInt &Isite) const |
Returns the matrix pointer to the \( S^+ \) operator at site Isite . More... | |
Mat | Sm (const PetscInt &Isite) const |
Returns the matrix pointer to the \( S^- \) operator at site Isite , if available. More... | |
const std::vector< Mat > & | Sz () const |
Returns the list of matrix pointer to the \( S^z \) operators. More... | |
const std::vector< Mat > & | Sp () const |
Returns the list of matrix pointer to the \( S^+ \) operators. More... | |
const std::vector< Mat > & | Sm () const |
Returns the list of matrix pointer to the \( S^- \) operators. More... | |
PetscErrorCode | CheckOperators () const |
Checks whether all operators have been initialized and have correct dimensions. More... | |
PetscErrorCode | CheckSectors () const |
Checks whether sector indexing was done properly. More... | |
PetscErrorCode | MatOpGetNNZs (const Op_t &OpType, const PetscInt &isite, std::vector< PetscInt > &nnzs) const |
Returns the number of non-zeros in each row for an operator acting on a given site. More... | |
PetscErrorCode | MatGetNNZs (const Mat &matin, std::vector< PetscInt > &nnzs) const |
Returns the number of non-zeros in each row for a given matrix. More... | |
PetscErrorCode | MatOpCheckOperatorBlocks (const Op_t &op_t, const PetscInt &isite) const |
Checks the block indexing in the matrix operator op_t on site isite. More... | |
PetscErrorCode | MatCheckOperatorBlocks (const Op_t &op_t, const Mat &matin) const |
Checks the block indexing in the matrix operator op_t on specified matrix matin. More... | |
PetscErrorCode | CheckOperatorBlocks () const |
Checks whether all matrix blocks follow the correct sector indices using MatCheckOperatorBlocks() More... | |
PetscErrorCode | GetOperatorBlocks (Op_t Operator) |
Extracts the block structure for each operator. More... | |
PetscErrorCode | CreateSm () |
Creates the Sm matrices on the fly. More... | |
PetscErrorCode | DestroySm () |
Destroys the Sm matrices on the fly. More... | |
PetscErrorCode | RotateOperators (SpinBase &Source, const Mat &RotMatT) |
Rotates all operators from a source block using the given transposed rotation matrix. More... | |
PetscErrorCode | AssembleOperators () |
Ensures that all operators are assembled. More... | |
Public Attributes | |
QuantumNumbers | Magnetization |
Stores the information on the magnetization Sectors. More... | |
Mat | H = nullptr |
Matrix representation of the Hamiltonian operator. More... | |
Protected Member Functions | |
PetscBool | MPIInitialized () const |
Returns PETSC_TRUE if the MPI communicator was initialized properly. More... | |
Private Types | |
enum | RotMethod { mmmmult =0, matptap =1 } |
List of possible methods for performing basis transformation. More... | |
Private Member Functions | |
PetscErrorCode | SaveOperator (const std::string &OpName, const size_t &isite, Mat &Op, const MPI_Comm &comm_in) |
Saves a single operator. More... | |
PetscErrorCode | Retrieve_NoChecks () |
Private function to retrieve operators without checking for save initialization. More... | |
Private Attributes | |
MPI_Comm | mpi_comm = PETSC_COMM_SELF |
MPI Communicator. More... | |
PetscMPIInt | mpi_rank |
MPI rank in mpi_comm. More... | |
PetscMPIInt | mpi_size |
MPI size of mpi_comm. More... | |
Spin_t | spin_type = SpinOneHalf |
Type of spin contained in the block. More... | |
std::string | spin_type_str = "1/2" |
Type of spin contained in the block expressed as a string. More... | |
PetscInt | _loc_dim = 2 |
Stores the local dimension of a single site. More... | |
std::vector< PetscScalar > | _loc_qn_list = {+0.5, -0.5} |
Stores the Sz sectors of a single site. More... | |
std::vector< PetscInt > | _loc_qn_size = {1, 1} |
Stores the number of states in each sector in a single site. More... | |
PetscBool | init = PETSC_FALSE |
Tells whether the block was initialized. More... | |
PetscBool | init_once = PETSC_FALSE |
Tells whether the block was initialized at least once before. More... | |
PetscBool | mpi_init = PETSC_FALSE |
Tells whether the block's MPI attributes were initialized. More... | |
PetscBool | verbose = PETSC_FALSE |
Tells whether to printout info during certain function calls. More... | |
PetscBool | log_io = PETSC_FALSE |
Tells whether to printout IO functions. More... | |
PetscInt | num_sites |
Number of sites in the block. More... | |
PetscInt | num_states |
Number of basis states in the Hilbert space. More... | |
PetscBool | init_Sm = PETSC_FALSE |
Tells whether the Sm matrices have been initialized. More... | |
std::vector< Mat > | SzData |
Array of matrices representing \(S^z\) operators. More... | |
std::vector< Mat > | SpData |
Array of matrices representing \(S^+\) operators. More... | |
std::vector< Mat > | SmData |
Array of matrices representing \(S^-\) operators. More... | |
PetscBool | init_save = PETSC_FALSE |
Whether saving the block matrices to file has been initialized correctly. More... | |
PetscBool | disk_set = PETSC_FALSE |
Whether SetDiskStorage() has properly set the block storage locations. More... | |
PetscBool | saved = PETSC_FALSE |
Whether the block matrices have been saved. More... | |
PetscBool | retrieved = PETSC_FALSE |
Whether the block matrices have been retrieved. More... | |
std::string | save_dir |
Root directory to read from and write the matrix blocks into. More... | |
std::string | read_dir |
Root directory to read the matrix blocks from during the first access. More... | |
std::string | write_dir |
Root directory to write the matrix blocks into. More... | |
PetscInt | num_reads = 0 |
Number of reads from file made for this block. More... | |
PetscInt | nsubcomm = 1 |
std::vector< PetscMPIInt > | site_color |
RotMethod | rot_method = mmmmult |
Method to use for performing basis transformation. More... | |
The documentation for this class was generated from the following files:
- include/DMRGBlock.hpp
- src/DMRGBlock.cpp